Interface vs Type Alias in TypeScript—Quick Comparison
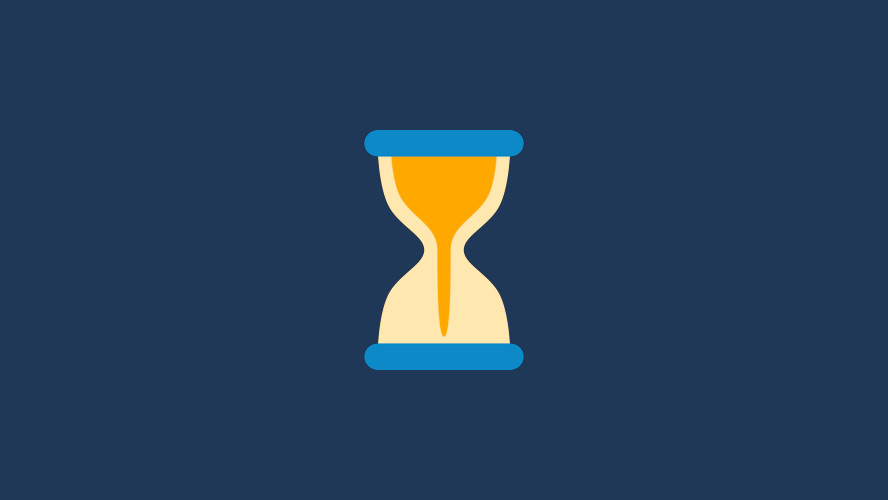
This can be confusing for anyone working with TypeScript—beginner or seasoned programmer. Both ways can cover similar needs.
In this post you’ll quickly see what Typescript feature is possible to implement as Type Alias or Interface. I stayed brief and spicy to give the post an overview character.
👉 TLDR: You don’t care about each difference? What should you use?
Use
interface
until you needtype
—orta
Overview: Interface (I) vs Type Alias (T)
Feature | I | T | Example |
---|---|---|---|
Primitives | ❌ | ✅ | type UUID = string |
Extend / Intersect | ✅ | ✅ | Response & ErrorHandling |
Unions | ❌ | ✅ | string | number |
Mapped object types | ❌ | ✅ | ['apple' | 'orange']: number |
Augment existing types | ✅ | ❌ | declare global { interface Window { … } } |
Declare type with typeof | ❌ | ✅ | Response = typeof ReturnType<fetch> |
Tuples | ✅ | ✅ | [string, number] |
Functions | ✅ | ✅ | (x: number, y: number): void |
Recursion | ✅ | ✅ | Nested { children?: Nested[] } |
Primitives
❌: Interface
✅: Type Alias
string
, number
and boolean
make up the Primitives in Typescript.
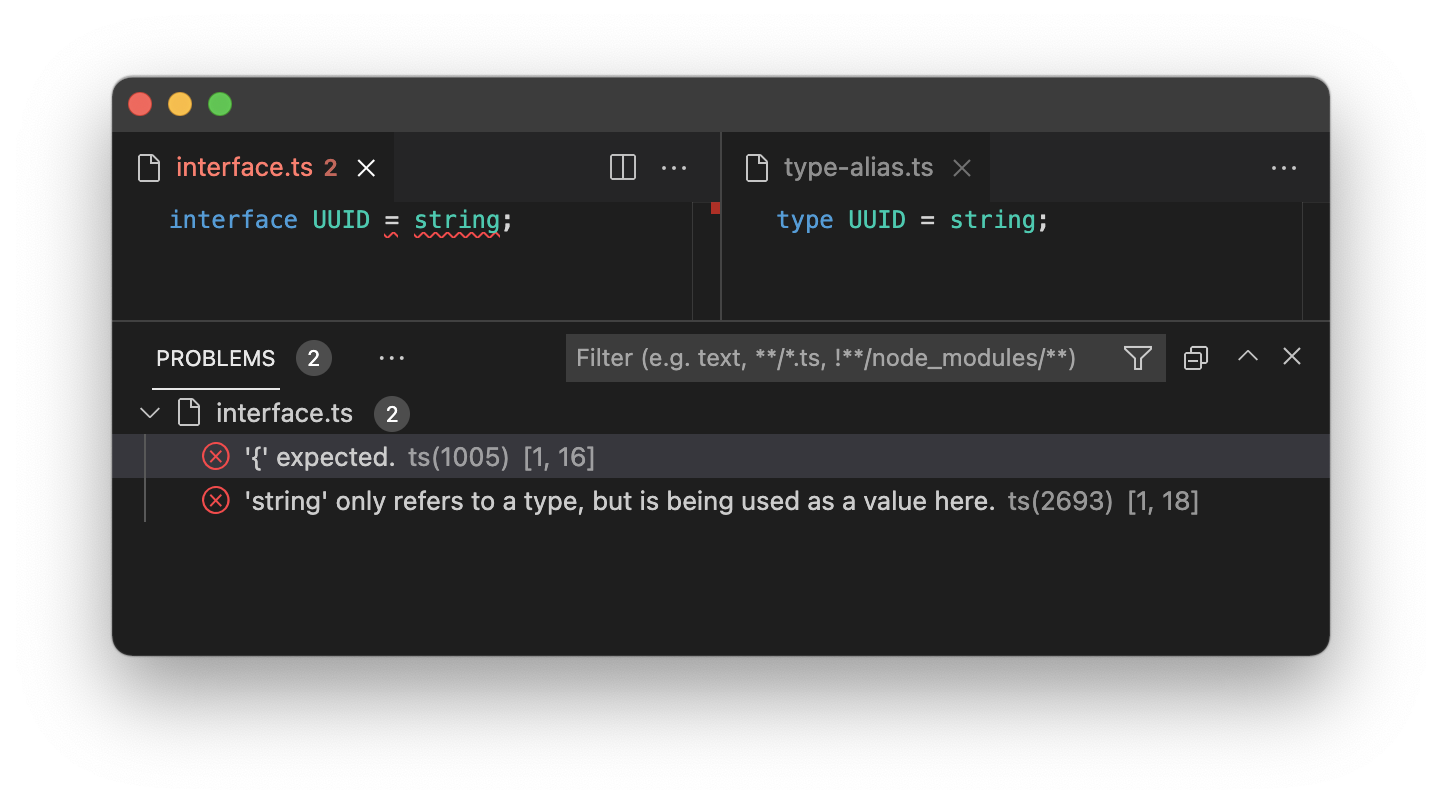
Extend / Intersect
✅: Interface
✅: Type Alias
Although intersect and extend are not 100% the same for interface and type alias, I put them together in this example. The differences arise when type keys appear in both types that you want to extend or intersect from.
So if the extended or intersected key is not the same type:
- Type Alias lets you do it but changes that type to
never
- Interface spits an error that the types are not compatible
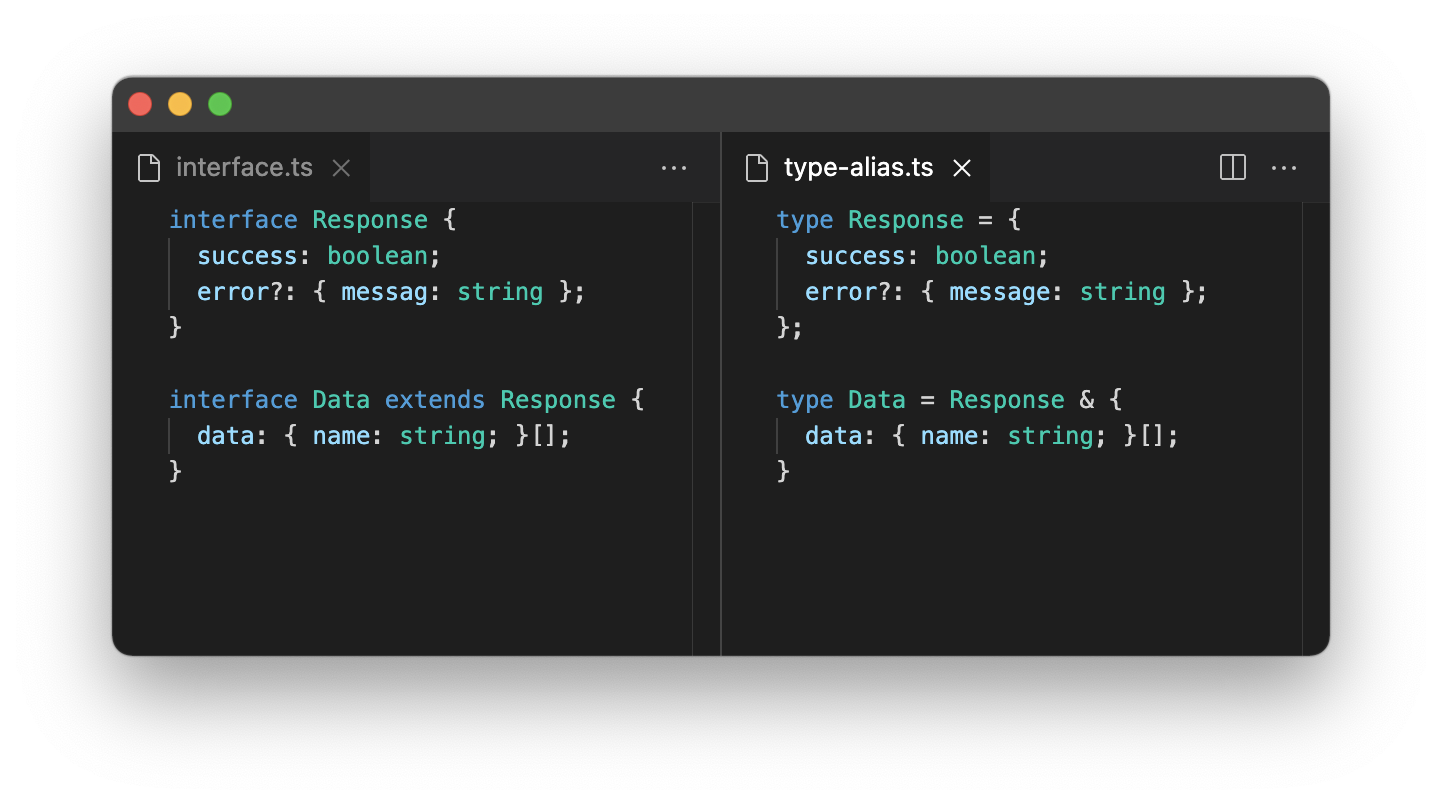
Mapped object types
❌: Interface
✅: Type Alias
Type the keys in your objects with this.
Here are a few useful applications of this:
[key: string]
: only strings as key allowed[key: number]
: only numbers as key allowed[key in keyof T as `get${Capitalize<string & key>}`]
: only allow keys that start withget...
, e.g. as seen in a Getter object
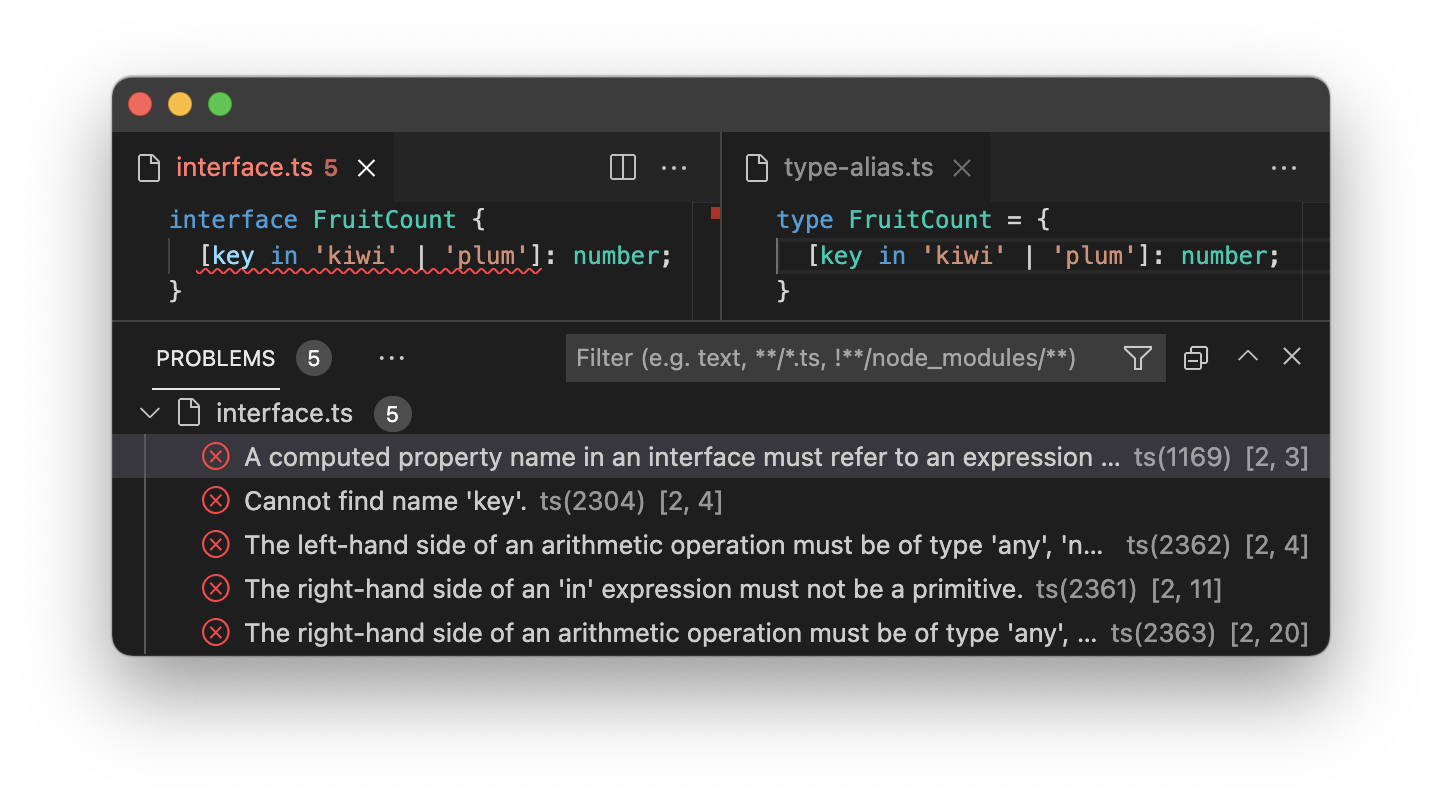
Unions
❌: Interface
✅: Type Alias
Typescript’s equivalent to OR
: The type is either x
or y
or z
or as many as you want.
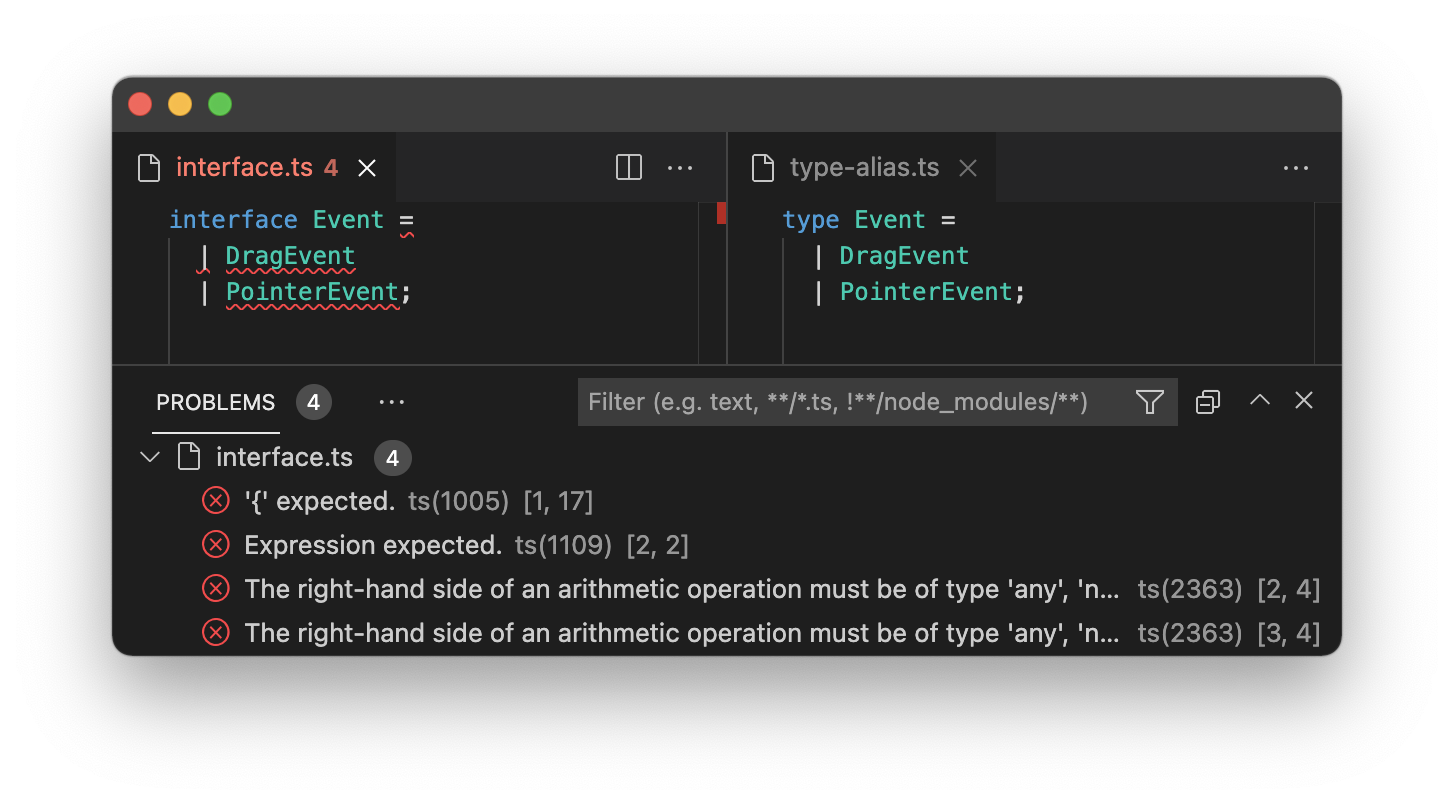
Augment existing types
✅: Interface
❌: Type Alias
You can add fields to already existing types. It’s useful when you add a new field (e.g. jQuery
library for auto completion) onto an existing type (e.g. window
).
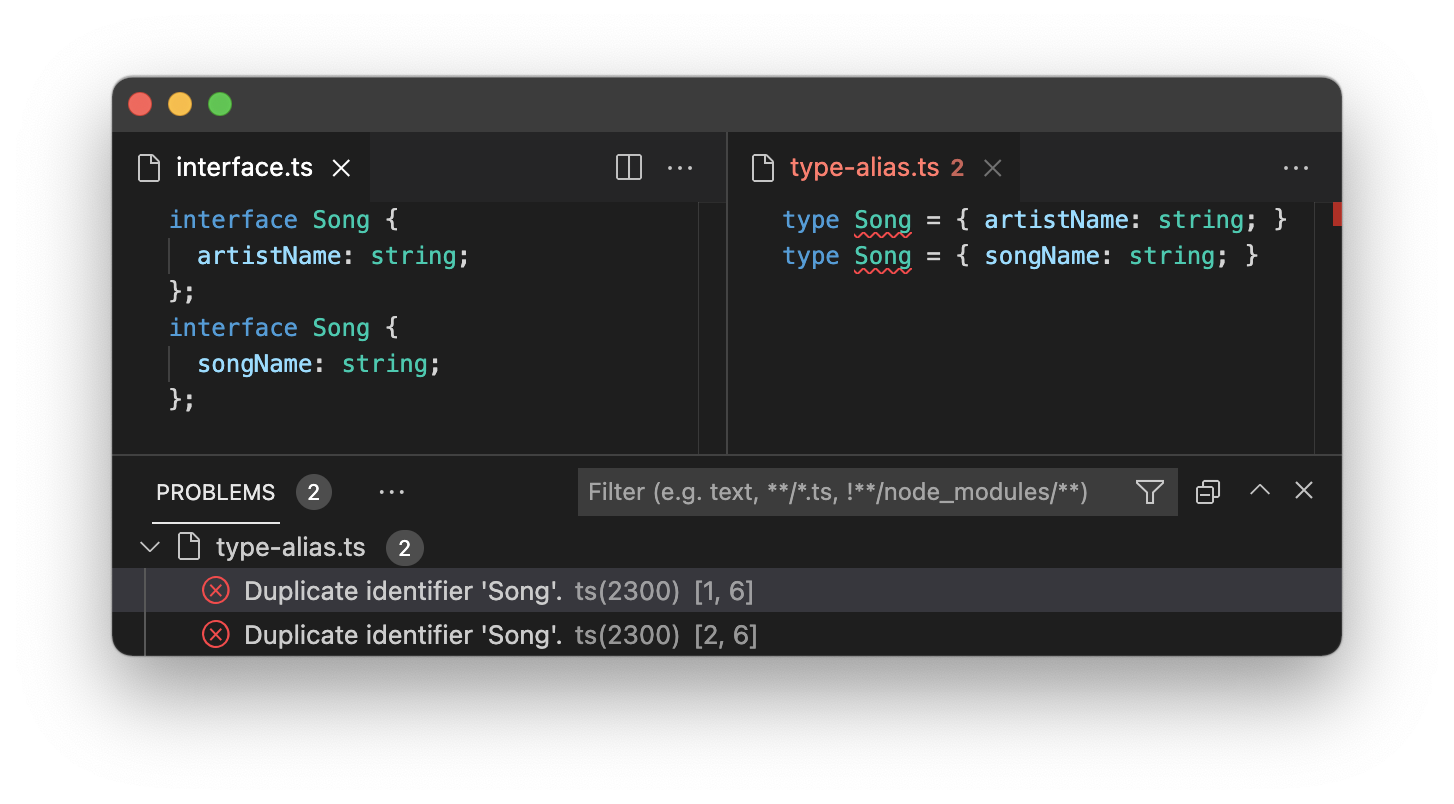
Tuples
✅: Interface
✅: Type Alias
If you’ve used hooks in react, then you know the usefulness of tuples.
A single function call can return an array of values and functions, that are destructured and can be used as fully typed variables: const [name, setName] = useState('')
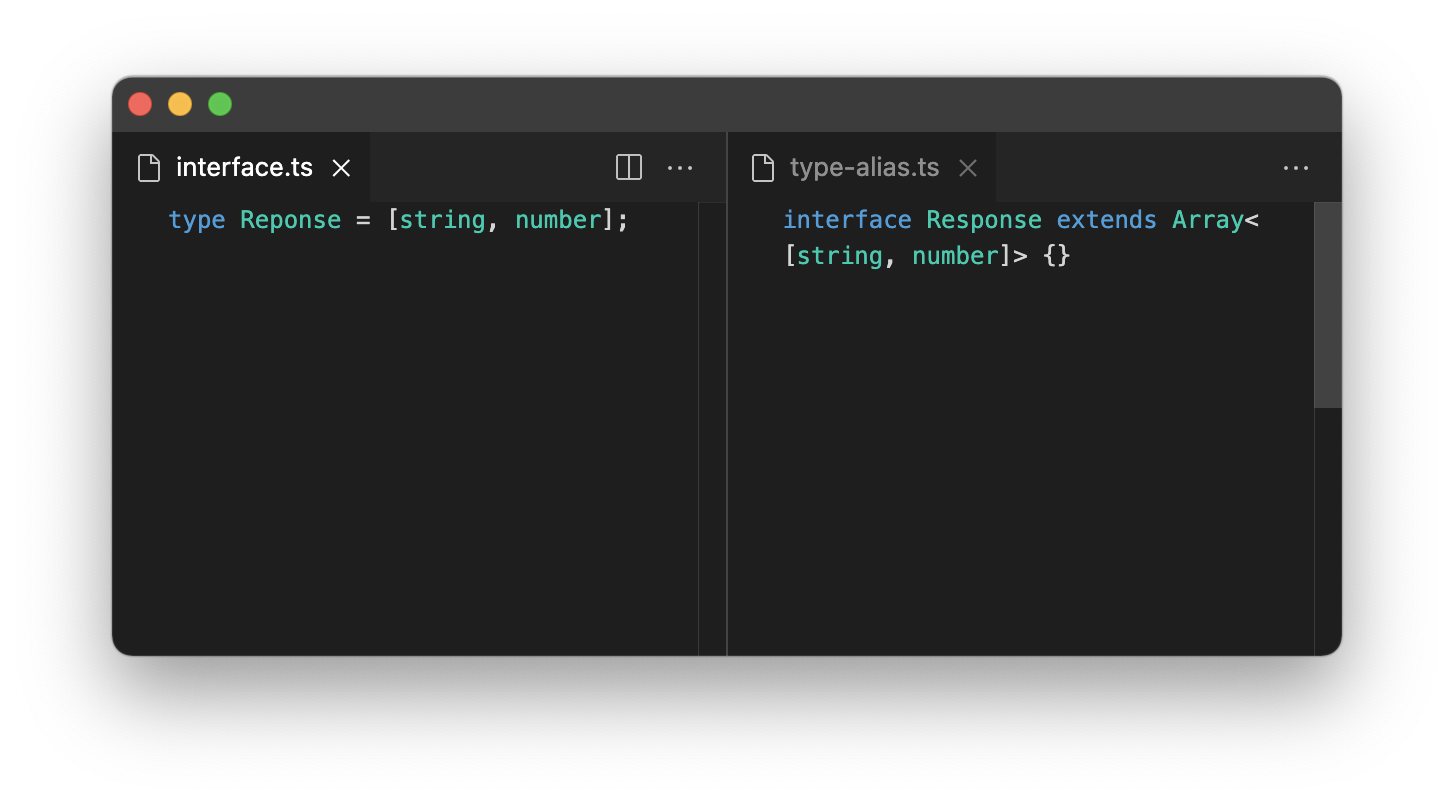
Functions
✅: Interface
✅: Type Alias
Functions can be annotated with Parameter Types and Return Types.
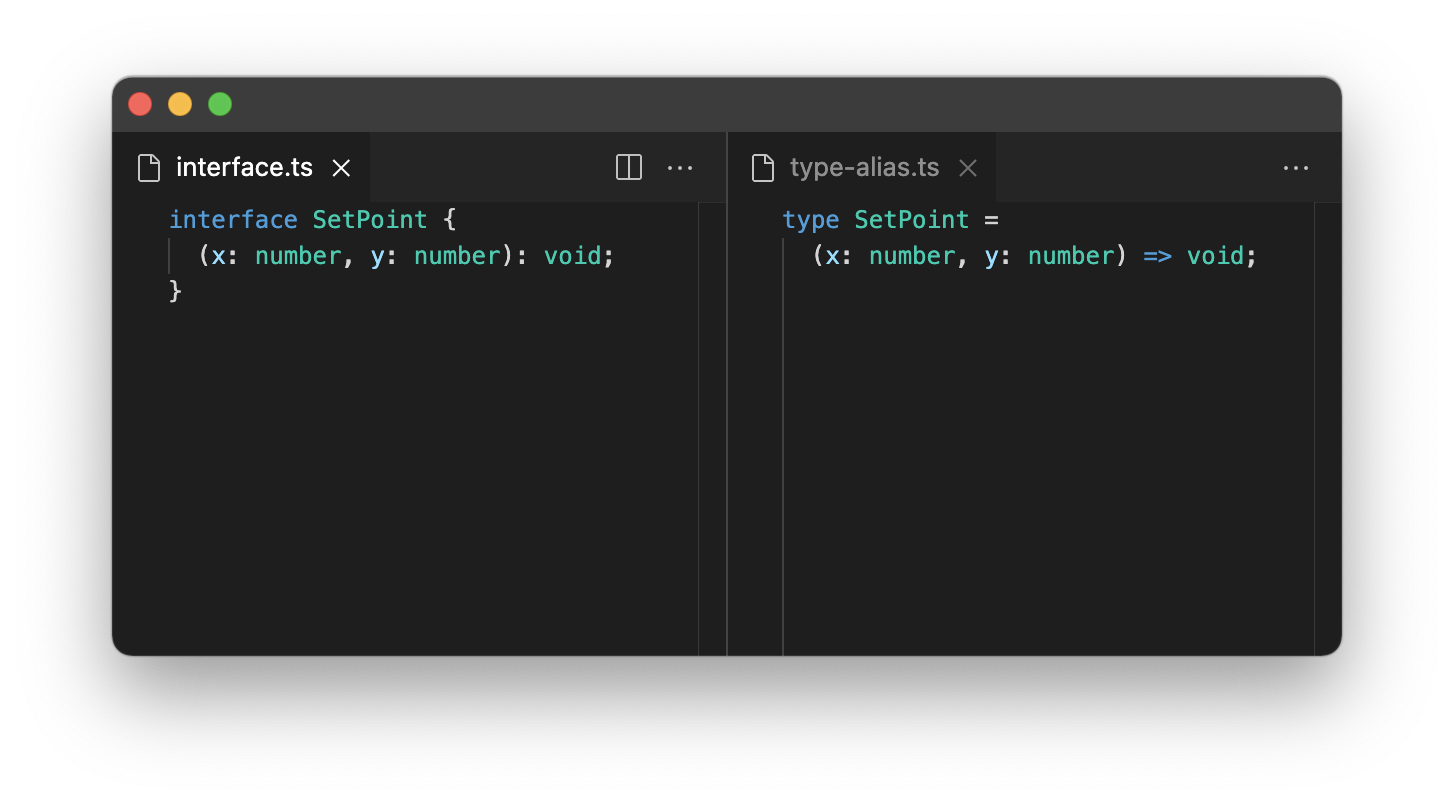
Recursion
✅: Interface
✅: Type Alias
Recursion are simple to use. Make sure you add the optional ?
to the recursive property. Otherwise the TS compiler spits out an error upon searching an endless recursion.
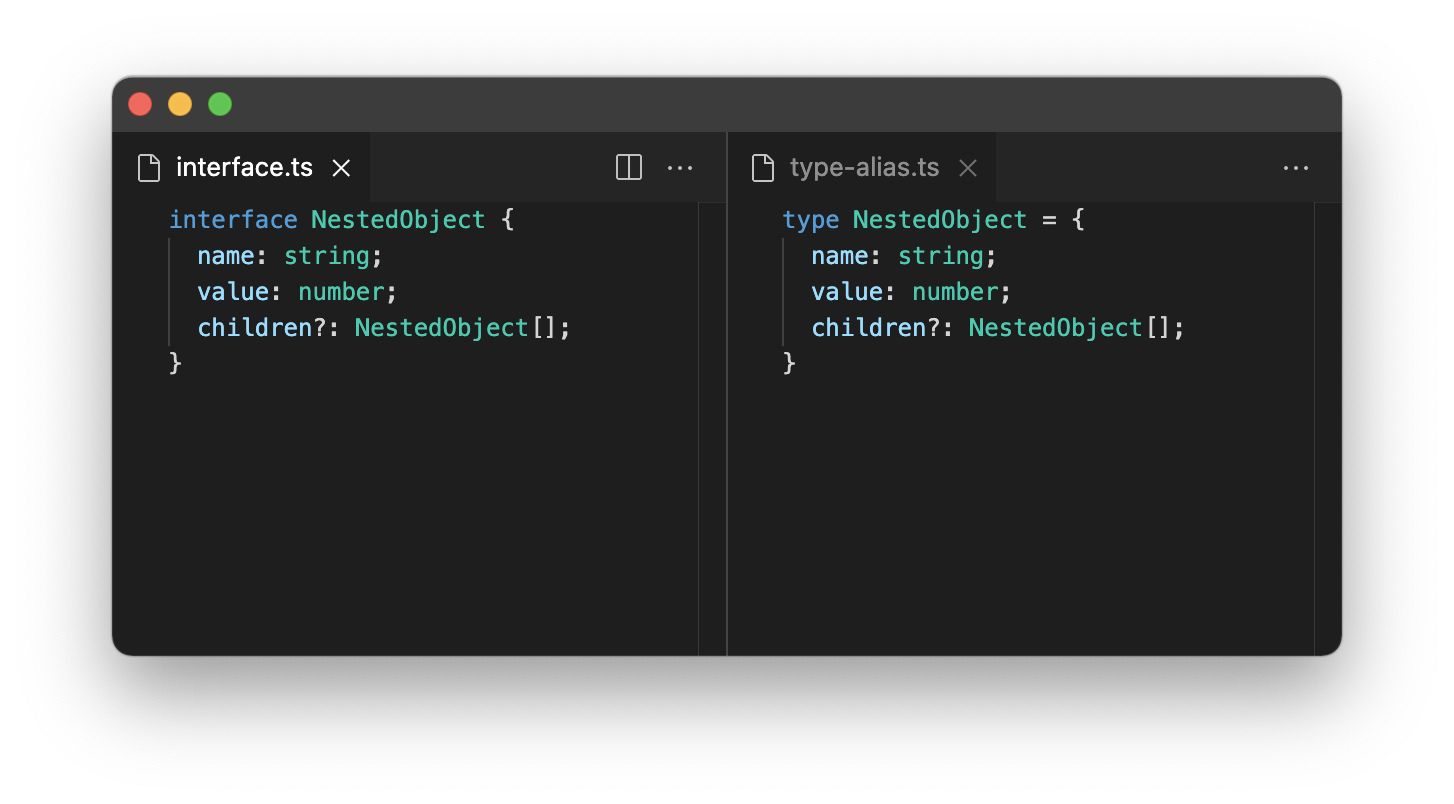
More resources
- Typescript Playground
- Types or Interfaces in react
- Interfaces vs Types in TypeScript by Mark
- Types vs. interfaces in TypeScript by Leonardo Maldonado
- Interface vs Type alias in TypeScript 2.7 by Martin Hochel
If you found this post interesting please leave a ❤️ on this tweet and consider following my 🎢 journey about #webperf, #buildinpublic and #frontend matters on Twitter.
Yall, let's talk about: type vs interface 🤔
— Simon Wicki (@zwacky) January 11, 2022
Ever wondered if you should use type (Type Alias) or interface in Typescript? 🤔
I put together a quick overview of TS features that you can or can't type with Type Aliases and Interfaces. [1/10] pic.twitter.com/2GQwrwnQ0u
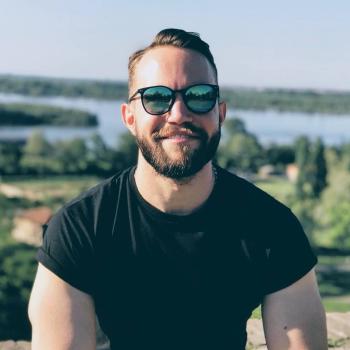
Simon Wicki is a Freelance Developer in Berlin. Worked on Web and Mobile apps at JustWatch. Fluent in Vue, Angular, React and Ionic. Passionate about Frontend, tech, web perf & non-fiction books.